반응형
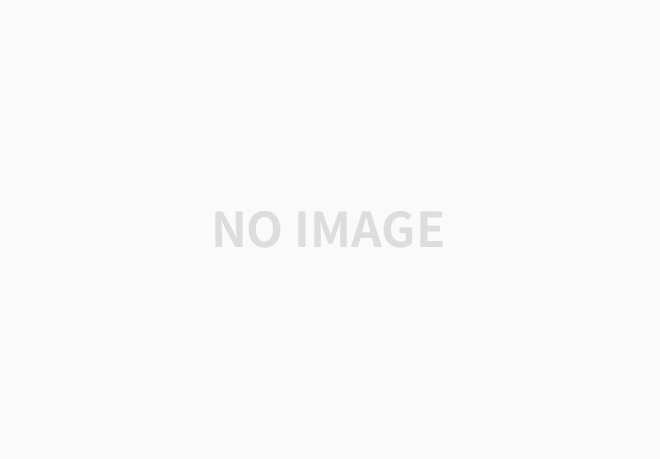
Minimum Path Sum - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
풀이
(0, 0)에서 출발하여 (m-1, n-1)까지 갈 때 grid에 적혀 있는 숫자의 합이 최솟값이 되는 경우를 찾는 문제. 간단하게 bfs를 사용해서 문제를 풀었는데 다른 사람들의 속도를 보니 dp를 활용하여 문제를 해결할 수 있는 것 같다. 추후에 dp에 대해서 공부할 때 다시 한 번 풀어봐야겠다.
코드
[Swift]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
|
typealias queueElement = (cost: Int, x: Int, y: Int)
class Solution {
func minPathSum(_ grid: [[Int]]) -> Int {
let m: Int = grid.count
let n: Int = grid[0].count
var ans: Int = Int.max
let dx: [Int] = [0, 1]
let dy: [Int] = [1, 0]
var flag: [[Bool]] = Array(repeating: Array(repeating: false, count: n), count: m)
var q: [queueElement] = [(grid[0][0], 0, 0)]
flag[0][0] = true
while !q.isEmpty {
q.sort(by: { $0.cost < $1.cost })
let qHead: queueElement = q.removeFirst()
if qHead.x == m-1 && qHead.y == n-1 {
ans = qHead.cost
break
}
for i in 0..<2 {
let nx: Int = qHead.x + dx[i]
let ny: Int = qHead.y + dy[i]
if nx >= m || ny >= n || flag[nx][ny] {
continue
}
q.append((qHead.cost + grid[nx][ny], nx, ny))
flag[nx][ny] = true
}
}
return ans
}
}
|
cs |
[Python]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
|
import heapq
class Solution(object):
def minPathSum(self, grid):
m = len(grid)
n = len(grid[0])
dx = [0, 1]
dy = [1, 0]
flag = [[False] * n for _ in range(m)]
q = []
heapq.heappush(q, (grid[0][0], 0, 0))
flag[0][0] = True
while True:
cost, x, y = heapq.heappop(q)
if x == m-1 and y == n-1:
return cost
for i in range(2):
nx = x + dx[i]
ny = y + dy[i]
if nx >= m or ny >= n or flag[nx][ny]:
continue
heapq.heappush(q, (cost+grid[nx][ny], nx, ny))
flag[nx][ny] = True
|
cs |
반응형
'Algorithm > LeetCode' 카테고리의 다른 글
[LeetCode] 778. Swim in Rising Water (Swift, Python) (0) | 2021.11.30 |
---|---|
[LeetCode] 841. Keys and Rooms (Swift, Python) (0) | 2021.11.29 |
[LeetCode] 1334. Find the City With the Smallest Number of Neighbors at a Threshold Distance (swift, python) (0) | 2021.11.12 |
[LeetCode] 743. Network Delay Time (swift, python) (0) | 2021.11.03 |
[LeetCode] 207. Course Schedule (swift, python) (0) | 2021.10.15 |
댓글