반응형
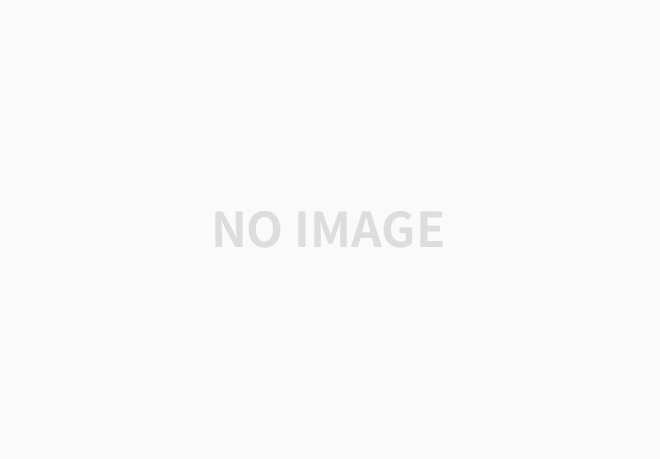
Network Delay Time - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
풀이
다익스트라 알고리즘을 활용해서 풀 수 있는 문제. 시작점 k에서 모든 노드까지의 최단거리를 구해준다. distance 배열에 k에서 각 노드 까지의 최단거리가 구해진다.
문제에서 모든 노드에 신호를 보내기까지 걸리는 시간을 구하라 했으므로 distance 배열에서 가장 큰 값(걸리는 시간이 가장 큰 경우)이 문제의 답이 된다. 만약 k에서 시작해서 도달할 수 없는 노드가 있을 경우에는 모든 노드에 신호를 보낼 수 없는 경우(impossible)이므로 -1을 리턴해주면 된다.
코드
[swift]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
|
typealias nodeTuple = (Int, Int)
class Solution {
func networkDelayTime(_ times: [[Int]], _ n: Int, _ k: Int) -> Int {
var graph = Array(repeating: [nodeTuple](), count: n+1)
var distance: [Int] = Array(repeating: Int(1e9), count: n+1)
for time in times {
graph[time[0]].append((time[1], time[2]))
}
var queue: [nodeTuple] = []
queue.append((0, k))
distance[k] = 0
while !queue.isEmpty {
queue.sort(by: { $0.0 > $1.0 })
let tuple: nodeTuple = queue.removeFirst()
let dist: Int = tuple.0
let now: Int = tuple.1
if distance[now] < dist {
continue
}
for g in graph[now] {
let cost: Int = dist + g.1
if cost < distance[g.0] {
distance[g.0] = cost
queue.append((cost, g.0))
}
}
}
var answer: Int = 0
for i in 1...n {
answer = max(answer, distance[i])
}
// 최단거리를 담는 배열에 INF와 같은 값이 있다면 k에서 모든 노드를 방문할 수 없다는 뜻이므로
// -1을 리턴
if answer == Int(1e9) {
return -1
}
return answer
}
}
let sol = Solution()
print(sol.networkDelayTime([[2,1,1],[2,3,1],[3,4,1]], 4, 2))
|
cs |
[python]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
|
pyimport heapq
INF = int(1e9)
class Solution:
def networkDelayTime(self, times: List[List[int]], n: int, k: int) -> int:
graph = [[] for _ in range(n+1)]
distance = [INF] * (n+1)
for (a, b, c) in times:
graph[a].append((b, c))
q = []
heapq.heappush(q, (0, k))
distance[k] = 0
while q:
dist, now = heapq.heappop(q)
if distance[now] < dist:
continue
for (node, nodeDist) in graph[now]:
cost = dist + nodeDist
if cost < distance[node]:
distance[node] = cost
heapq.heappush(q, (cost, node))
answer = 0
for i in range(1, n+1):
answer = max(answer, distance[i])
# 최단거리를 담는 배열에 INF와 같은 값이 있다면 k에서 모든 노드를 방문할 수 없다는 뜻이므로
# -1을 리턴
if answer == INF:
return -1
return answer
|
cs |
반응형
'Algorithm > LeetCode' 카테고리의 다른 글
[LeetCode] 64. Minimum Path Sum (Swift, Python) (0) | 2021.11.16 |
---|---|
[LeetCode] 1334. Find the City With the Smallest Number of Neighbors at a Threshold Distance (swift, python) (0) | 2021.11.12 |
[LeetCode] 207. Course Schedule (swift, python) (0) | 2021.10.15 |
[LeetCode] 200. Number of Islands (swift) (0) | 2021.10.13 |
[LeetCode] 797. All Paths From Source to Target (swift) (0) | 2021.10.13 |
댓글