반응형
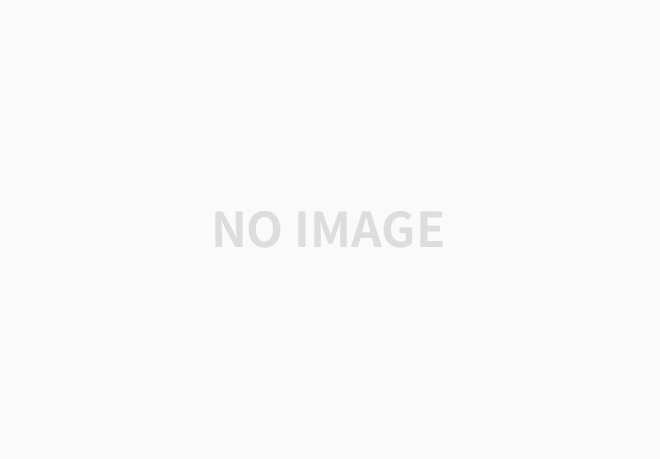
코딩테스트 연습 - 프린터
일반적인 프린터는 인쇄 요청이 들어온 순서대로 인쇄합니다. 그렇기 때문에 중요한 문서가 나중에 인쇄될 수 있습니다. 이런 문제를 보완하기 위해 중요도가 높은 문서를 먼저 인쇄하는 프린
programmers.co.kr
풀이
총 3개의 스택/큐를 만들었다.
- 인쇄해야 하는 큐(a) : 인쇄해야 하는 문서들이 들어있는 큐
- 인쇄 순서를 담아두는 큐(b) : 우선순위에 따른 인쇄 순서를 담아두는 큐.
- 임시 pool(c) : b에서 우선순위에 밀려 다음으로 미뤄진 리스트를 임시로 저장하여 a에 넣어주기 위해 사용되는 큐
a는 입력으로 주어진 인쇄 리스트를 의미한다. 차례대로 앞에서부터 b에 담아주는데 만약 b에 가장 뒤쪽에 있는 문서에 비해 우선순위가 높은지 낮은지 체크한다. 만약 b에 있는 문서가 우선순위가 낮을 경우 임시로 c에 담아준다. 이를 반복한 후 현재 문서를 b에 넣어주면 c에 임시로 담아두었던 문서들을 다시 a에 삽입하여 이와 같은 과정을 반복한다.
코드
[Swift]
import Foundation
typealias printTuple = (idx: Int, priority: Int)
var printStack: [printTuple] = []
// 현재 문서보다 우선순위가 낮은 문서들을 다시 printList에 담아주기 위한 함수
func stackToPool(_ priority: printTuple) -> [printTuple] {
var poolQueue: [printTuple] = []
// 현재 우선순위보다 낮은 문서들을 pool에 임시로 담아주고 현재 문서를 printStack에 넣어준다.
while !printStack.isEmpty && printStack[printStack.count - 1].priority < priority.priority {
poolQueue.insert(printStack.removeLast(), at: 0)
}
printStack.append(priority)
return poolQueue
}
func solution(_ priorities:[Int], _ location:Int) -> Int {
var answer: Int = 0
var printList: [printTuple] = [] // 인덱스 정보를 포함한 인쇄해야 하는 문서들의 리스트
for i in 0..<priorities.count {
printList.append((i, priorities[i]))
}
// printStack에 아직 문서가 없는 경우에는 바로 넣어준다.
// 그렇지 않을 경우 우선순위를 따져 현재 문서보다 우선순위가 낮은 경우는
// 다시 printList에 담을 수 있도록 한다.
while !printList.isEmpty {
let priority: printTuple = printList.removeFirst()
if printStack.count == 0 {
printStack.append(priority)
} else {
printList += stackToPool(priority)
}
}
// printStack의 앞에서부터 location을 만날때까지 반복한다.
for stack in printStack {
answer += 1
if stack.idx == location {
break
}
}
return answer
}
[Python]
printStack = []
# 현재 문서보다 우선순위가 낮은 문서들을 다시 printList에 담아주기 위한 함수
def stackToPool(priority):
poolQueue = []
# 현재 우선순위보다 낮은 문서들을 pool에 임시로 담아주고 현재 문서를 printStack에 넣어준다.
while len(printStack) != 0 and printStack[-1][1] < priority[1]:
poolQueue.insert(0, printStack.pop())
printStack.append(priority)
return poolQueue
def solution(priorities, location):
answer = 0
# 인덱스 정보를 포함한 인쇄해야 하는 문서들의 리스트
printList = []
for priority in enumerate(priorities):
printList.append(priority)
# printStack에 아직 문서가 없는 경우에는 바로 넣어준다.
# 그렇지 않을 경우 우선순위를 따져 현재 문서보다 우선순위가 낮은 경우는
# 다시 printList에 담을 수 있도록 한다.
while printList:
priority = printList.pop(0)
if len(printStack) == 0:
printStack.append(priority)
else:
printList += stackToPool(priority)
# printStack의 앞에서부터 location을 만날때까지 반복한다.
for (idx, _) in printStack:
answer += 1
if idx == location:
break
return answer
반응형
'Algorithm > Programmers' 카테고리의 다른 글
[고득점 Kit(스택/큐)] 주식가격 (Python) (0) | 2022.01.19 |
---|---|
[고득점 Kit(스택/큐)] 다리를 지나는 트럭 (Swift, Python) (0) | 2022.01.18 |
[고득점 Kit(스택/큐)] 기능개발 (Swift, Python) (0) | 2022.01.15 |
[고득점 Kit(탐욕법)] 단속카메라 (Python) (0) | 2022.01.03 |
[고득점 Kit(탐욕법)] 섬 연결하기 (Swift, Python) (0) | 2022.01.02 |
댓글